The object-oriented programming paradigm is prevalent in all modern tools and languages for creating software. The industry standard today is the object-oriented programming language C ++. You can create an instance of a class in C ++ in several different ways.
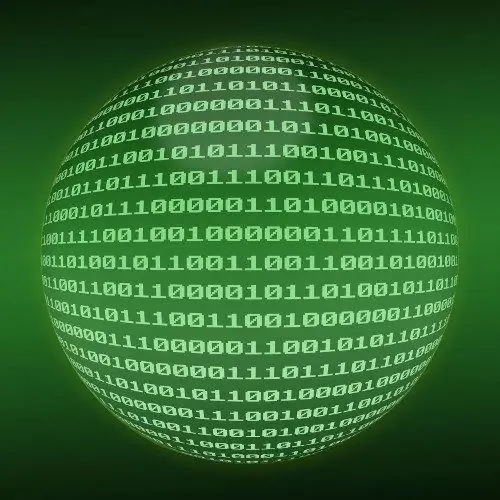
It is necessary
C ++ compiler
Instructions
Step 1
Instantiate the class as an auto variable in the local scope defined by a function, class method, or statement block. Use a declarative or imperative definition of a class object at a selected location in your program. If necessary, make an explicit call to any constructor with parameters. Create an object using code similar to the following: void CMyClass:: SomeMethod () {COtherClass oSomeObject1; // create an object using the default constructor COtherClass oSomeObject2 (1980, "Victor V. Vakchturov"); // creating an object using a constructor with parameters} Memory for objects of classes created in a similar way, as for any other auto-variables, is allocated on the stack. Therefore, when you exit the scope and remove the stack frame, the object will be destroyed (with a call to the destructor).
Step 2
Create an instance of the class in the heap using the new operator. Define a variable of type pointer to objects of the class to be instantiated. Give it a value that is the result of evaluating the new operator. Call the appropriate constructor. Use a code snippet similar to the following: CSomeClass * poSomeObject; // definition of a pointer to objects of the class CSomeClasspoSomeObject = new CSomeClass; // Create an object of class CSomeClass * poSomeObject_2 = new CSomeClass (111, "3V"); // creation with a call to the constructor with parameters When creating objects by this method, the memory allocation mechanism defined by the new operator is used (if it is not overridden and its own allocation function is not set), so the address of the new object is not known in advance. All objects created in this way must be explicitly deleted using the delete operator.
Step 3
Create an instance of the class using the new operator on a self-allocated chunk of memory. Use code similar to the following: void * p0 = malloc (sizeof (CSomeClass)); // memory allocation void * p1 = malloc (sizeof (CSomeClass)); // memory allocation new (p0) CSomeClass; // initialize the object on the allocated memory (default constructor) new (p1) CSomeClass (111, "abc"); // object initialization (constructor with parameters) Before destroying objects created by this method, you should explicitly call their destructor: ((CSomeClass *) p0) -> ~ (); Creating objects in this way is mainly used in template container classes of various libraries (such as STL).