Finding the largest or smallest value in an array is a very common task in programming. And since the most common programming languages in relation to the Internet today are the server-side PHP language and the client-side JavaScript language, below are several options for solving this problem for these languages.
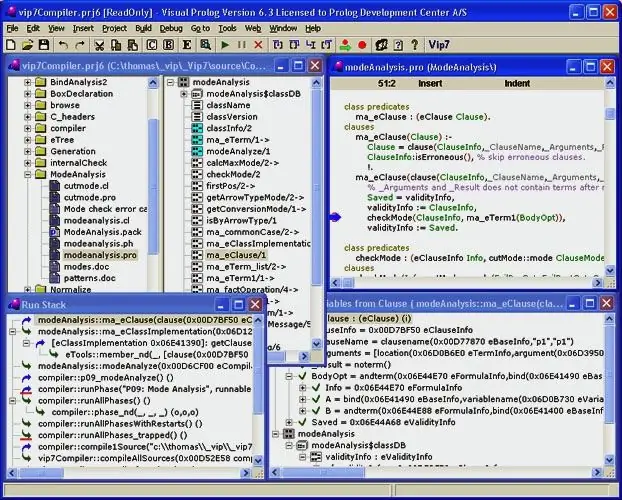
Instructions
Step 1
Organize the iteration over all the elements of the array, comparing the value of each subsequent one with the previous one and remembering the maximum value in a separate variable. In PHP, the corresponding block of code may look, for example, like this - first, define an array: $ values = array (14, 25.2, 72, 60, 3); Then assign a separate variable the value of the first element - it will be considered the maximum before the iteration begins: $ maxValue = $ values [0]; Organize a loop comparing the previously stored value with the current one. Remember or skip the current value based on the comparison results: foreach ($ values as $ val) if ($ val> $ maxValue) $ maxValue = $ val; Print the detected maximum value:
echo $ maxValue;
Step 2
In JavaScript, the same algorithm can be implemented, for example, with the following code:
var values = [14, 25.2, 72, 60, 3];
var maxValue = values [0]
for (var i = 1; i <= values.length-1; i ++) {
if (values > maxValue) maxValue = values ;
}
alert (maxValue);
Step 3
However, there is no need to organize the check yourself, since most programming languages have built-in functions that will do this for you. For example, in PHP, you can use the rsort function to sort the elements of an array in descending order. The corresponding code for the array used in the first step might look like this: <? Php
$ values = array (14, 25.2, 72, 60, 3);
rsort ($ values);
echo $ values [0];
?>
Step 4
For JavaScript, the easiest way is to use the max method of the Math object by passing an array as an argument to it using another method, applay. For example, with the following code: var values = [14, 25.2, 72, 60, 3];
alert (Math.max.apply ({}, values))